
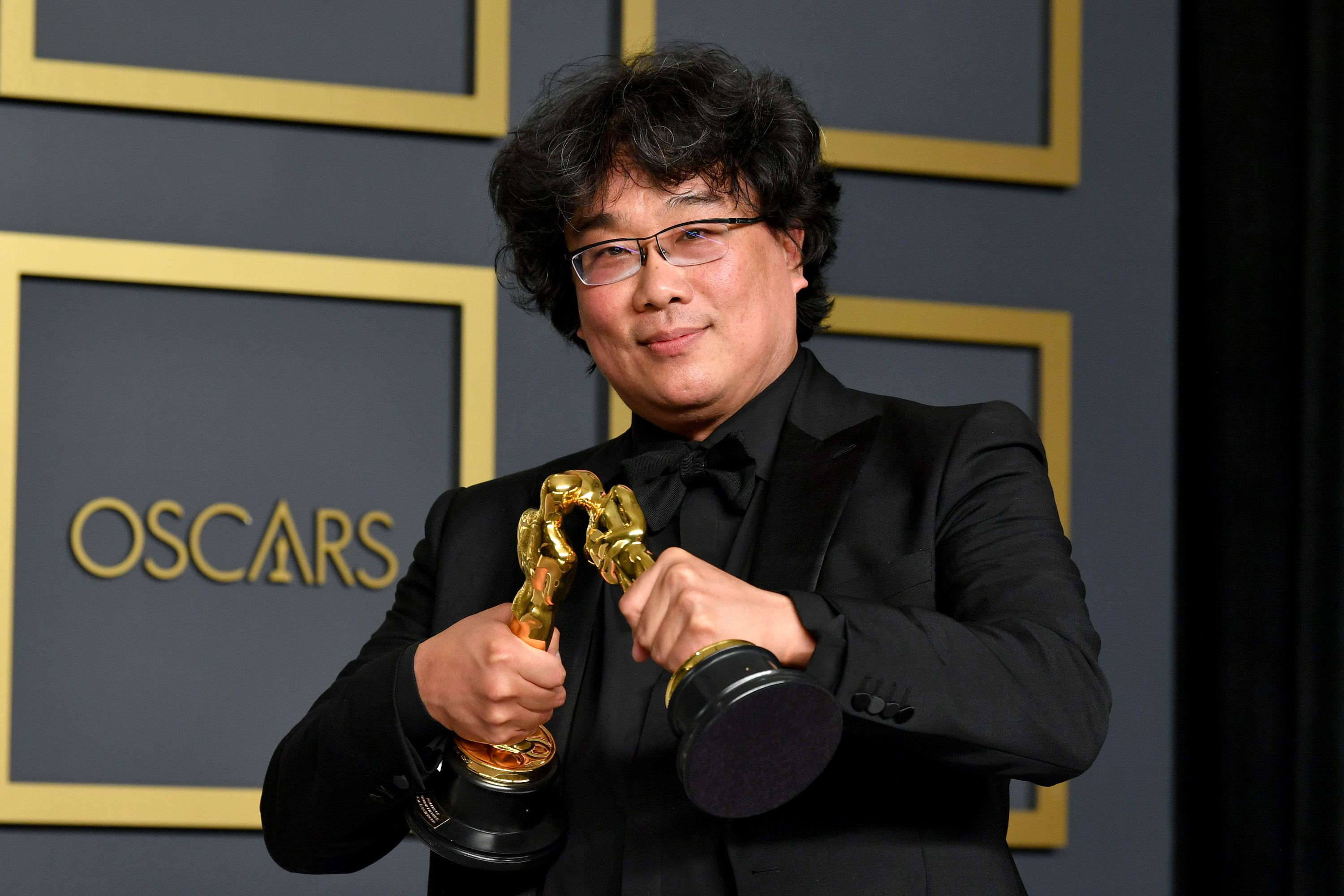
Yeah, but Friends was on Netflix early and most people I know watched it on there during college
Yeah, but Friends was on Netflix early and most people I know watched it on there during college
I’m trying my best
Ugh. It just devolves into “China 1984 Tianamem Uighur” like 2/3rds of the way though right after saying “US users broke out of their echo chamber”
Not bad for a side hustle in the States and not bad for a main hustle if your bilingual and live in a country with a reasonable cost of living
You did, I was just batch responding to everyone that got pinged by the bot report
I was just passing the message along lol, leaving those in just means they know a bunch of people from Hexbear followed your link
Apparently xiaohongshu has tracking tokens in the link copy feature and a bot here is upset about it lol
I got a bot report because you left tracking tokens in your links, maybe remove them? (It’s everything after the question mark)
Yeah, but if an AI spots them it won’t find any and the problem is solved
I can kinda see their point, as in every 2nd and 3rd frame will be an estimate from DLSS instead of actually pushing the vertexes through the render pipeline. But the use of brute force definitely does something funny painting their major selling point as brutish to sell a minor feature.
To be fair, it is probably the least actually exploitative form of pornography, while also being the most ethically exploitative at the same time and for the same reasons.
That old group never went away, they just got drowned out by market forces
I was interested in the methods used!
Did you freehand or use a vinyl cutout?
The bands are good, there’s also the Nothing CMF watch which is alright. I think the Xiaomi band has better tracking, but the CMF watch does have some pretty watchface options.
They’re from another instance, AFAIK our mods don’t care about that aspect, and only care about limiting critical support for possible fascists.
Framework is neat because you can buy the components from them, but S76 and Thinkpad are good because they’re easy to take apart and you can usually just slap off the shelf components on them as long as the main board still works.
Some older Thinkpads even have socketed CPUs.
It’s kinda dumb when all this could just be nationalized and run under one umbrella, but MNO and MVNO leasing is at least better than having to pay roaming charges because Verizon or AT&T don’t have ownership of the Bell infrastructure in your region because that’s how the cards fell when they broke it up.
It crashes on me all the time in Windows 11 too